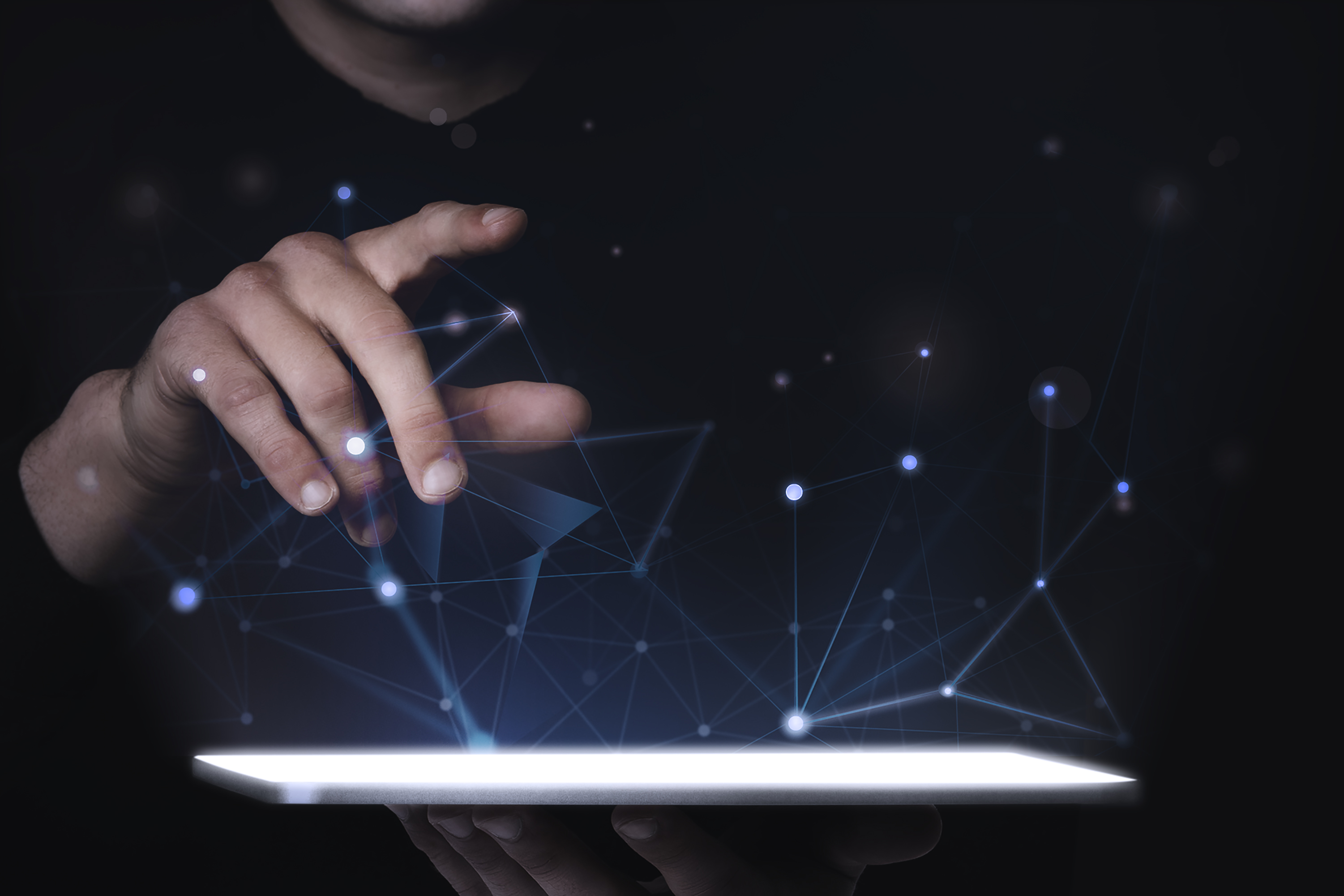
JavaScript concept is a high-level, dynamic, multi-paradigm programming language used for web development, creating interactive user interfaces, and building server-side applications. It was created in 1995 by Brendan Eich while he was working at Netscape Communications Corporation. Today, it is one of the most popular programming languages in the world, with a large and active developer community.
Oops is used in JavaScript and it is a client-side language, meaning that it runs in the browser and can interact with HTML and CSS to create dynamic and responsive web pages. It can also be used on the server side, with Node.js, to create powerful and scalable back-end applications.
JavaScript is an interpreted language, meaning that it doesn’t need to be compiled before running. It is also dynamically typed, meaning that variables can hold different types of data at different times during execution. JavaScript supports functional, object-oriented, and imperative programming paradigms. This article will help you understand JavaScript fundamentals and code better.
Here is the list of the top 8 advanced concepts of JavaScript that you must know before starting a project. These advanced concepts will help JavaScript developers to understand this language better.
Closures are a powerful concept in JavaScript that allows you to create private variables and methods inside a function. It is achieved by creating a function inside another function and returning it. Here is an example:
function outerFunction() {
var outerVar = “I am outer”; function innerFunction() { var innerVar = “I am inner”; console.log(outerVar, innerVar); } return innerFunction; } var inner = outerFunction(); inner(); |
Promises are a way to handle asynchronous operations in JavaScript. Promises are objects that represent the eventual completion or failure of an asynchronous operation and allow you to attach callbacks to them. Here is an example:
function getData() {
return new Promise((resolve, reject) => { setTimeout(() => { resolve(“Data fetched successfully!”); }, 2000); }); } getData().then((data) => { console.log(data); }); |
Async/await is a modern syntax for handling asynchronous operations in JavaScript. Async/await makes it easier to write asynchronous code in a synchronous way. Here is an example:
function getData() {
return new Promise((resolve, reject) => { setTimeout(() => { resolve(“Data fetched successfully!”); }, 2000); }); } async function main() { const data = await getData(); console.log(data); } main(); |
Generators are functions that can be paused and resumed at any point. Generators are defined using the function* keyword and the yield keyword is used to pause the function. Here is an example:
function* generatorFunction() {
yield 1; yield 2; yield 3; } const generator = generatorFunction(); console.log(generator.next().value); console.log(generator.next().value); console.log(generator.next().value); |
Proxy is a powerful feature in JavaScript that allows you to intercept and customize the behavior of objects. Proxy is defined using the Proxy object and a handler object is passed to it. Here is an example:
const person = {
name: “John”, age: 30, }; const handler = { get: function (target, prop) { console.log(`Getting ${prop} property`); return target[prop]; }, set: function (target, prop, value) { console.log(`Setting ${prop} property to ${value}`); target[prop] = value; }, }; const proxy = new Proxy(person, handler); proxy.name; proxy.age = 40; console.log(person); |
Maps are a new data structure introduced in ES6 that allows you to store key-value pairs. Unlike objects, maps can use any type of key, not just strings. Here is an example:
const map = new Map();
map.set(“name”, “John”); map.set(“age”, 30); console.log(map.get(“name”)); console.log(map.get(“age”)); |
Sets are another new data structure introduced in ES6 that allows you to store unique values. Here is an example:
const set = new Set();
set.add(1); set.add(2); set.add(3); console.log(set.has(1)); console.log(set.has(4)); |
The spread operator is a syntax for spreading the elements of an array or object into another array or object. Here is an example:
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6]; const arr3 |
The best part about learning the advanced concepts of JavaScript is that it enables you to build an application more user-friendly and interactive. JavaScript is considered the best programming language for building an advance and user-friendly web application.
The post Important JavaScript concepts that every web developer must know appeared first on .
Client satisfaction is our ultimate goal. Here are some kind words of our precious clients they have used to express their satisfaction with our service.
I came across Adequate Infosoft while searching for an IT company to design a virtual platform for my Telemedicine business. AI helped me to make my dream project a reality.
The price and professionalism of Adequate Infosoft's project team are the most appealing aspects of working with them. The team provides weekly progress reports and responds quickly to the concerns I have.
My team is very satisfied with the professionalism shown by the Adequate Infosoft team during the project. We are looking forward to working with them again.
The team at Adequate understood our requirements very well and delivered everything on time. And the resultant solutions were better than what we expected. We will surely look forward to working with them again in the near future.
I contacted AI for an Android and iOS application and I am completely satisfied with their service.
I am very satisfied with Adequate Infosoft. very helpful, positive, and quick communication so far. I am looking forward to further cooperation.
Great experience hiring them, understood the requirements very well, and were very effective and efficient in delivering the project. I will hire them for my next project as well and also recommend them to others.
Adequate Infosoft lead development team is efficient and provides the best IT solutions. If you're looking for quick and affordable software development, Adequate Infosoft is your go-to guru!
Adequate Infosoft has stood out to be the best company for providing IT services at affordable prices. Their rapid development approach works in line with our iterative process.
We have worked with Adequate Infosoft for 4 years and it has been a positive experience for me and my company.
Adequate Infosoft has set a benchmark with its robust product development services. Their development team is highly professional that understands the value of time.
Exceptional service! The AI team guided me through the entire procedure and made it an enjoyable experience.
As a small business, we were most attracted to Adequate Infosoft's competitive pricing and the ability to quickly scale up or down the number of developers supporting the application.
It was a pleasure to collaborate with Adequate Infosoft. Their development team is comprised of true experts.
Send your message in the form below and we will get back to you as early as possible.
Captcha is required
Leave a Reply